AI_Node Class Reference
[Utilities]
#include <AI_Node.h>
Inheritance diagram for AI_Node:
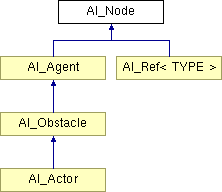
Detailed Description
Implement a node in a doubly linked list.
- Version:
- 2.0 AI_Node was ripped from The Nebula Device and renamed to AI_Node. Now it is possible to use the class inside and outside Nebula projects.
Public Member Functions | |
AI_Node () | |
the default constructor | |
AI_Node (void *ptr) | |
constructor providing user data pointer | |
~AI_Node () | |
the destructor | |
AI_Node * | GetSucc () const |
get the next node in the list | |
AI_Node * | GetPred () const |
get the previous node in the list | |
void | InsertBefore (AI_Node *succ) |
insert this node before 'succ' node into list | |
void | InsertAfter (AI_Node *pred) |
insert this node after 'pred' node into list | |
void | Remove () |
remove node from list | |
void | SetPtr (void *p) |
set user data pointer | |
void * | GetPtr () const |
get user data pointer | |
bool | IsLinked () const |
check if node is currently linked into a list | |
Friends | |
class | AI_List |
Constructor & Destructor Documentation
AI_Node::AI_Node | ( | void | ) | [inline] |
the default constructor
AI_Node::AI_Node | ( | void * | ptr | ) | [inline] |
constructor providing user data pointer
AI_Node::~AI_Node | ( | void | ) | [inline] |
the destructor
The destructor will throw an assertion if the node is still linked into a list!
Member Function Documentation
AI_Node * AI_Node::GetSucc | ( | void | ) | const [inline] |
get the next node in the list
Get the node after this node in the list, return 0 if there is no next node.
- Returns:
- the next node or 0
AI_Node * AI_Node::GetPred | ( | void | ) | const [inline] |
get the previous node in the list
Get the node before this node in the list, return 0 if there is no previous node.
- Returns:
- the previous node or 0
void AI_Node::InsertBefore | ( | AI_Node * | succ | ) | [inline] |
insert this node before 'succ' node into list
- Parameters:
-
succ node in front of which this node should be inserted
void AI_Node::InsertAfter | ( | AI_Node * | pred | ) | [inline] |
insert this node after 'pred' node into list
- Parameters:
-
pred the node after which this node should be inserted
void AI_Node::Remove | ( | void | ) | [inline] |
remove node from list
void AI_Node::SetPtr | ( | void * | p | ) | [inline] |
set user data pointer
- Parameters:
-
p the new user data pointer
void * AI_Node::GetPtr | ( | ) | const [inline] |
get user data pointer
- Returns:
- the user data pointer
bool AI_Node::IsLinked | ( | void | ) | const [inline] |
check if node is currently linked into a list
- Returns:
- true if node is currently linked into a list
Friends And Related Function Documentation
friend class AI_List [friend] |
The documentation for this class was generated from the following file: