AI_Agent Class Reference
[InExIn]
#include <AI_Agent.h>
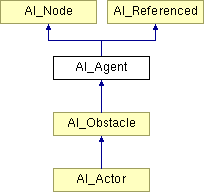
Detailed Description
Agent in the AI world.
Agent is a simpliest representation of an object in AI world. It is also base class for more complicated AI agents.
Agent has its position and orientation in the world. Whenever the position will be changed the move() method must be called. The orientation is in euler angles.
The agent has also so-called "aura". Aura is a space aroud each agent which demarks agent's presence in the spatial database. In fact it is a bounding box which is considered by spatial database. Aura is set automatically so you do not need to set manually in most cases.
- Version:
- 1.0 Initial release.
Public Types | |
enum | TYPE { AGENT, OBSTACLE, ACTOR } |
Type of an agent. More... | |
typedef AI_Array< AI_Agent * > | AgentArray |
Array of agent pointers. | |
typedef AgentArray::iterator | AgentArrayIt |
Iterator of an array of agents. | |
Public Member Functions | |
AI_Agent (void) | |
Constructor. | |
AI_Agent (const TYPE type) | |
Constructor with type (do not call). | |
~AI_Agent () | |
Destructor. | |
void | setPosition (const AI_Vector3 &v_position) |
Set position of the agent. | |
void | setOrientation (const AI_Vector3 &v_orientation) |
Set orientation of the agent in euler angles. | |
void | setAura (const AI_Vector3 &v_aura) |
Set aura as an extents around center of an agent (see clas desc.). | |
void | setSpatialElement (AI_SDB_BBoxSpatialElement *ptr_element) |
Set spatial element in spatial database (it is not for user usage). | |
void | setWorld (AI_World *ptr_world) |
Set pointer to the AI_World into which the agent belongs (used by AI_World). | |
void | setUserData (void *ptr_user_data) |
Set pointer to user data. | |
virtual void | setEnable (const bool b_enable) |
Enable/disable agent. | |
const TYPE & | getType (void) const |
Return type of an agent (one type for once class). | |
const AI_Vector3 & | getPosition (void) const |
Get agent's position. | |
const AI_Vector3 & | getOrientation (void) const |
Get orientation of the agent in euler angles. | |
const AI_Vector3 & | getAura (void) const |
Get aura of an agent (see class desc.). | |
AI_SDB_BBoxSpatialElement * | getSpatialElement (void) |
Get spatial element (it is not for user usage). | |
const bool | isEnable (void) const |
Is agent enabled? | |
AI_World * | getWorld (void) |
Get pointer to the AI_World into which the agent belongs (used by AI_World). | |
void * | getUserData (void) |
Get pointer to user data. | |
AgentArray & | getNearAgents (const float f_distance) |
const float | getDistance (const AI_Vector3 &v_position) const |
Compute and return distance of this agent from given position. | |
const bool | isInRange (const AI_Agent *ptr_agent, const float f_range) const |
Return true if given position is in given range from this agent. | |
void | move (void) |
Should be called whenever the position of an agent will change. | |
Protected Attributes | |
TYPE | m_type |
Type of agent. | |
AI_Vector3 | m_v_position |
Position of center of an agent. | |
AI_Vector3 | m_v_orientation |
Orientation in euler angles. | |
AI_World * | m_ptr_world |
World this agent belongs to. | |
bool | m_b_enable |
Agent is enabled (it is considered in world operations). | |
AI_Vector3 | m_v_aura |
Lenghts of bbox that represents agent in spatial database. | |
AI_SDB_BBoxSpatialElement * | m_ptr_spatial_element |
Element of spatial database. | |
void * | m_ptr_user_data |
User data. |
Member Typedef Documentation
typedef AI_Array<AI_Agent*> AI_Agent::AgentArray |
Array of agent pointers.
Iterator of an array of agents.
Member Enumeration Documentation
enum AI_Agent::TYPE |
Constructor & Destructor Documentation
AI_Agent::AI_Agent | ( | void | ) | [inline] |
Constructor.
AI_Agent::AI_Agent | ( | const TYPE | type | ) | [inline] |
Constructor with type (do not call).
AI_Agent::~AI_Agent | ( | ) |
Destructor.
Member Function Documentation
void AI_Agent::setPosition | ( | const AI_Vector3 & | v_position | ) | [inline] |
Set position of the agent.
void AI_Agent::setOrientation | ( | const AI_Vector3 & | v_orientation | ) | [inline] |
Set orientation of the agent in euler angles.
void AI_Agent::setAura | ( | const AI_Vector3 & | v_aura | ) | [inline] |
Set aura as an extents around center of an agent (see clas desc.).
void AI_Agent::setSpatialElement | ( | AI_SDB_BBoxSpatialElement * | ptr_element | ) | [inline] |
Set spatial element in spatial database (it is not for user usage).
void AI_Agent::setWorld | ( | AI_World * | ptr_world | ) | [inline] |
void AI_Agent::setUserData | ( | void * | ptr_user_data | ) | [inline] |
Set pointer to user data.
This data are not used in any way by AI library.
void AI_Agent::setEnable | ( | const bool | b_enable | ) | [inline, virtual] |
Enable/disable agent.
Agent is enabled only when it is part of any world.
Reimplemented in AI_Actor.
const AI_Agent::TYPE & AI_Agent::getType | ( | void | ) | const [inline] |
Return type of an agent (one type for once class).
const AI_Vector3 & AI_Agent::getPosition | ( | void | ) | const [inline] |
Get agent's position.
const AI_Vector3 & AI_Agent::getOrientation | ( | void | ) | const [inline] |
Get orientation of the agent in euler angles.
const AI_Vector3 & AI_Agent::getAura | ( | void | ) | const [inline] |
Get aura of an agent (see class desc.).
AI_SDB_BBoxSpatialElement * AI_Agent::getSpatialElement | ( | void | ) | [inline] |
Get spatial element (it is not for user usage).
const bool AI_Agent::isEnable | ( | void | ) | const [inline] |
Is agent enabled?
Enabled / disabled agents are considered only within world triggering and only when the agent is an actor.
AI_World * AI_Agent::getWorld | ( | void | ) | [inline] |
void * AI_Agent::getUserData | ( | void | ) | [inline] |
Get pointer to user data.
This data are not used in any way by AI library.
AgentArray& AI_Agent::getNearAgents | ( | const float | f_distance | ) |
Reimplemented in AI_Actor.
const float AI_Agent::getDistance | ( | const AI_Vector3 & | v_position | ) | const [inline] |
Compute and return distance of this agent from given position.
const bool AI_Agent::isInRange | ( | const AI_Agent * | ptr_agent, | |
const float | f_range | |||
) | const [inline] |
Return true if given position is in given range from this agent.
void AI_Agent::move | ( | void | ) |
Should be called whenever the position of an agent will change.
Member Data Documentation
TYPE AI_Agent::m_type [protected] |
Type of agent.
AI_Vector3 AI_Agent::m_v_position [protected] |
Position of center of an agent.
AI_Vector3 AI_Agent::m_v_orientation [protected] |
Orientation in euler angles.
AI_World* AI_Agent::m_ptr_world [protected] |
World this agent belongs to.
bool AI_Agent::m_b_enable [protected] |
Agent is enabled (it is considered in world operations).
AI_Vector3 AI_Agent::m_v_aura [protected] |
Lenghts of bbox that represents agent in spatial database.
AI_SDB_BBoxSpatialElement* AI_Agent::m_ptr_spatial_element [protected] |
Element of spatial database.
void* AI_Agent::m_ptr_user_data [protected] |
User data.
The documentation for this class was generated from the following files: