AI_Actor Class Reference
[InExIn]
#include <AI_Actor.h>
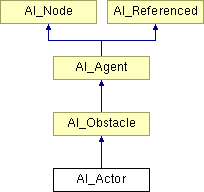
Detailed Description
AI agent as an actor.Actor is representation of any character who wants to be driven by the state machine and/or navigated by pathfinding and who wants to avoid any static or dynamic obstacle.
Actor must be triggered in each frame to work properly. Please ensure the actor was added into any AI world before it is triggered. Once the actor is in AI world then it is triggered by AI_World::trigger() method.
If you wish to drive your actor by the state machine (SM) then create the SM object and add several states from AI_Actor's internal states into the SM. Then set this SM into the actor. If the AI is turned off (default) or SM was not set then the SM will not work within acotr's triggering. If the SM was set and AI is turned on then the SM will work once per period given by reflex value (if the reflex is 0.2 (default) then the state machine will work five times per second).
Pathfinding is performed everytime when
- the state machine decides (without user assitance)
- so called "reflective behaviour" decides (without user assitance)
- the user decides and calls walkTo() or chaseActor()
Frequency of the pathfinding replaning is optimized and it is as low as possible.
Reflective behaviour means low level decisioning such as near visible obstacle avoidance. To avoid near small objects (obstacles) the actor uses so-called feeler. Feeler looks like a tentacle and is used to feel surround straight before the actor. Feeler can be updated automatically (default) and in this case the length of the feeler is set to feel surround on the way for one second. Feeler length can be set by user.
Actor uses so-called pivot. Pivot is point od the path (path found by pathfinder) and is moved by same linear velocity as the actor itself. Actor allways try to go directly towards the pivot except cases when he is outside the path because of some obstacle before him. Distance of the pivot can be set automatically (to be one and half second from the actor) or by user.
The actor works in asynchronous way. It means you can put the actor into any state by several asynchronous commands (walkTo(), chaseActor(), turnTo() etc.) or by turning the AI on (in this case the states are changing automaticaly). Then you can read what the actor is actualy doing and you must also submit any feedback to him.
Actor can acting exactly one act at one moment. Some acts require any kind of the feedback to work properly:
- If agent is in TURN act then he needs per frame updating of its orientation.
- If agent is in WALK act then he needs per frame updating of its position and orientation.
- If agent once becom into ATTACK act then ATTACK_FINISHED feedback must be sent to the agent when attack is finished (typically at the end of one attack animation).
The actor never move or turn itself. It must be done outside of the AI library (for example in your physical engine). First read actual AI actor's acting and then read following aditional informations:
- If agent is in TURN act then read turn direction.
- If agent is in WALK act then read walk direction and turn direction.
- If agent is in ATTACK then read attack target.
The most important characteristic of the actor is "reflex". It means:
- delay between two state machine decisioning (call of AI_StateMachine::work())
- minimum delay between two get near agents/obstacles/items/actors (the array of near agents is cached)
Actor's visibility is used mainly by StateIsSee for visibility determination.
Here are examples of actor's state machines.
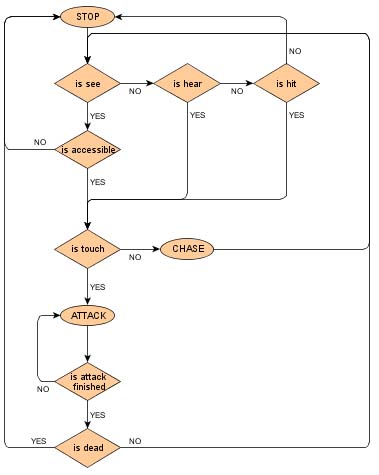
Close range attacker
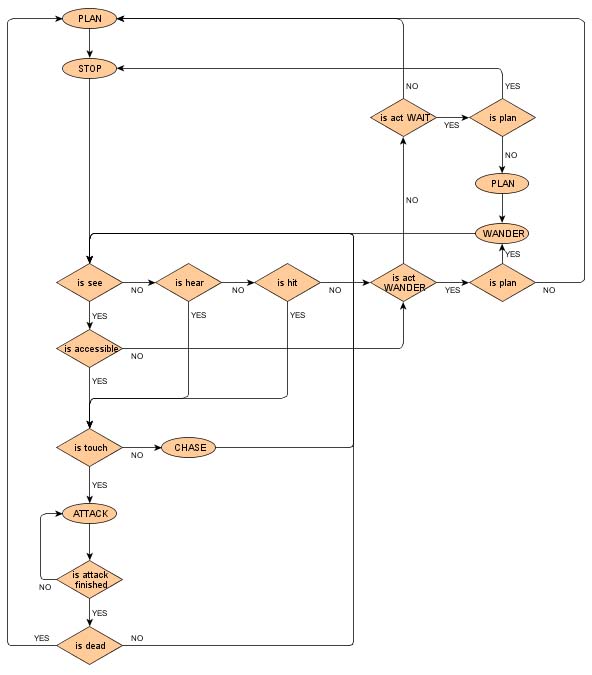
Wandering close range attacker
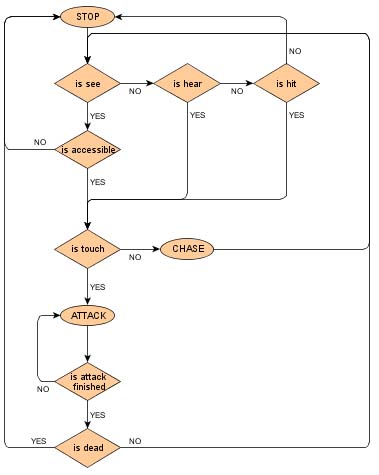
Long range attacker
- Version:
- 1.3 (8.8.2006)
- StateIsDead was removed
- StateIsAct was added
- StateTimePlan was added
- StateIsTimePlan was added
1.2 (7.8.2006)
- Wandering (wander() command and StateWander added)
1.1 (10.5.2006)
- Actor's visiblity was added.
- Actor's noise was added.
- Locked Actor was divided into the Locked Chased and Locked Enemy
- Enemy type was added (hero, other clans, particular clan)
- These states was modified according to all previous changes: StateAttack, StateChase, StateIsAccessible, StateIsHear, StateIsInOptimum, StateIsInRange, StateIsSee, StateIsTouch
1.0 Initial release.
Public Types | |
enum | ACT { NONE, WAIT, WALK, WANDER, TURN, ATTACK, DEATH } |
Actors actual act. More... | |
enum | FEEDBACK { ATTACK_FINISHED = (1<<0), HIT = (1<<1) } |
Feedback from outer world. More... | |
enum | ENEMY_TYPE { ET_HERO, ET_OTHER_CLANS, ET_CLAN } |
Who is an enemy for the actor? More... | |
typedef AI_Array< AI_Actor * > | ActorArray |
Array of actor pointers. | |
typedef ActorArray::iterator | ActorArrayIt |
Iterator of an array of actors. | |
Public Member Functions | |
AI_Actor (void) | |
Default constructor. | |
~AI_Actor (void) | |
Destructor. | |
void | trigger (double d_time) |
Trigger actor's behaviour in one frame. | |
void | setLockedChased (AI_Actor *ptr_actor) |
Lock actor for chasing. | |
AI_Actor * | getLockedChased (void) |
Get actor locked for chasing. | |
void | setLockedEnemy (AI_Actor *ptr_actor) |
Lock an enemy. | |
void | setAttacker (AI_Actor *ptr_attacker) |
Set an actor who attack this actor. | |
AI_Actor * | getLockedEnemy (void) |
Get locked enemy. | |
AI_Actor * | getAttacker (void) |
Get attacker. | |
bool | updateNearAgents (const float f_distance) |
Build array of agents that are nearer then given distance. | |
AgentArray & | getNearAgents (const float f_distance) |
Return foreign agents in given range around this actor. | |
ActorArray & | getNearActors (const float f_distance) |
Return foreign actors in given range around this actor. | |
ObstacleArray & | getNearObstacles (const float f_distance) |
Return foreign obstacles in given range around this actor. | |
ActorArray & | getNearEnemies (const float f_distance) |
const bool | isEnemy (AI_Actor *ptr_actor) |
Return true if given actor is an enemy for this actor. | |
virtual void | setEnable (const bool b_enable) |
Enable actor. | |
const AI_Vector3 & | getDestination (void) |
Return destination set previously by walkTo(). | |
AI_NavigationMesh::Session & | getNavmeshSession (void) |
Get navigation mesh session. | |
void | clearWay (void) |
Clear any founded way. | |
int | getWaySize (void) const |
Number of waypoints on the way. | |
AI_Vector3 & | getWayPoint (int i) const |
Get way point on the way (must be between 0 and way size). | |
const bool | isTouch (AI_Obstacle *ptr_agent_other, const float f_tolerance) const |
Is actor touching another actor with given tolerance? | |
const bool | isCollision (AI_Obstacle *ptr_obstacle, const double d_time) const |
Will the actor collide with given obstacle in given time? | |
const AI_Vector3 & | getWalkDirection (void) const |
Get vector of desired walking. | |
const AI_Vector3 & | getWalkWayPoint (void) const |
Get actual way point. | |
const AI_Vector2 & | getFeelerTip (void) const |
Get feeler tip 2d position (for debug visualisation purposes). | |
const AI_Vector3 & | getPivot (void) const |
Get way pivot (for debug visualisation purposes). | |
const float | getTurnDirection (void) const |
Get turn direction. | |
const float | getDesiredAngle (void) const |
Return desired angle. | |
const float | getWayLength (void) |
Overal length of the way. | |
bool | isOnSurface (void) |
Is actor over surface of navigation mesh? | |
bool | getNearestSurfacePosition (AI_Vector3 &v_nearest_position) |
Return nearest position on navigation mesh to actor within range of his width. | |
void | setAIEnable (bool b_ai=true) |
Enable/disable actor. | |
bool | isAIEnable (void) const |
Is AI enabled? | |
void | setStateMachine (AI_StateMachine *ptr_state_machine) |
Set state machine which will drive this actor. | |
AI_StateMachine * | getStateMachine (void) |
Get state machine. | |
AI_StateMachine::Session & | getStateMachineSession (void) |
Get state machine session. | |
void | setAct (int i_act) |
Set actual actor's acting. | |
int | getAct (void) const |
Return actual actor's acting. | |
const AI_Actor * | getAttackTarget (void) |
const bool | isSleeping (void) const |
Return true if actor is sleeping (due to collision with another actor). | |
void | setFeelerLength (bool b_fixed, float f_length=-1) |
Set feeler length. | |
void | setPivotDistance (bool b_fixed, float f_distance=-1) |
Set pivot distance. | |
const float | getFeelerLength (void) const |
Get feeler length. | |
const float | getPivotDistance (void) const |
Get pivot distance. | |
void | setRefex (const double d_reflex) |
Set time between two actor's reactions (in second). | |
void | setLinearVelocity (const float f_linear_velocity) |
Set linear velocity. | |
void | setAngularVelocity (const float f_angular_velocity) |
Set angular velocity. | |
void | setClan (const int i_clan) |
Set clan into which the actor belongs. | |
void | setEnemyClan (const int i_clan) |
Set enemy clan. | |
void | setEnemyType (const ENEMY_TYPE type) |
Set who is enemy for this actor. | |
void | setSight (const float f_sight) |
Set maximum distance to what the actor can see other actors. | |
void | setEarshot (const float f_earshot) |
Get maximum distance to what the actor can hear other actors. | |
void | setFOV (const float f_fov) |
Set field of view (in radians). | |
void | setRange (const float f_range) |
Set maximum distance for ranged attack. | |
void | setOptimalDistance (const float f_dist) |
Set optimal distance for attack. | |
void | setChaseDistance (const float f_dist) |
Set maximum distance for chasing. | |
virtual void | setWidth (const float f_width) |
Set width of the actor. | |
void | setVisible (const bool b) |
Set actor's visibility. | |
void | setNoise (const bool b) |
Set whether actor is performing some noise. | |
void | setUntouchable (const bool b) |
Get pivot distance. | |
const float | getAngularVelocity (void) const |
Get angular velocity. | |
const double | getReflex (void) const |
Get reflex (in seconds). | |
const float | getLinearVelocity (void) const |
Get linear velocity of an actor. | |
const int | getClan (void) const |
Get clan into which the actor belongs. | |
const int | getEnemyClan (void) const |
Get enemy clan. | |
const ENEMY_TYPE | getEnemyType (void) const |
Who is enemy for this actor? | |
const float | getSight (void) const |
Get maximum distance to what the actor can see other actors. | |
const float | getEarshot (void) const |
Get distance to what the acto hears other actors. | |
const float | getFOV (void) const |
Get field of view angle in radians. | |
const float | getRange (void) const |
Get maximum possible distance for attack. | |
const float | getOptimalDistance (void) const |
Get optimal distance for attack. | |
const float | getChaseDistance (void) const |
Get max chasing distance. | |
const bool | isVisible (void) const |
Is actor visible? | |
const bool | isNoise (void) const |
Is actor performing some noise? | |
const bool | isUntouchable (void) const |
Get pivot distance. | |
void | walkTo (const AI_Vector3 &v_dest) |
Walk to given position. | |
void | wander (void) |
Wander. | |
void | turnTo (const float f_angle) |
Turn to given angle. | |
void | chaseActor (AI_Actor *ptr_actor) |
Chase an actor. | |
void | attack (AI_Actor *ptr_actor) |
Attack another actor. | |
void | stop (void) |
Instantly stops the actor whatever he is doing. | |
void | die (void) |
Kill actor. | |
void | reset (void) |
Reset actor. | |
void | sendFeedback (FEEDBACK dw_feedback) |
Send feedback to this actor. | |
bool | checkFeedback (ai_dword dw_feedback) |
Ask for particualar sent feedback. | |
void | clearFeedback (ai_dword dw_feedback) |
Clear particular feedback. | |
void | flushFeedback (void) |
Clear all feedbacks. | |
Protected Member Functions | |
void | updateWait (const double d_time) |
Update waiting. | |
void | updateWalkDirection (const double d_time) |
Update actor while he is walking. | |
void | updateWandering (const double d_time) |
Update actor while wandering. | |
void | updateOrientation (const double d_time) |
Update actor's orientation while actor is just turning. | |
void | updateAttack (const double d_time) |
Update attacking. | |
bool | findWay (void) |
Instantly find way and return true if way was found. | |
void | updatePivot (const double d_time) |
Return foreign items in given range around this actor. | |
void | updateFeeler (void) |
Update feeler tip position. | |
void | updateDestination (const double d_time) |
Update destination while some actor is chased. | |
AI_Obstacle * | findNearestObstacle (const double d_time, float &f_obstacle_distance) |
Examine space before actor by feeler and return nearest feeled obstacle. | |
float | findNearestWall (float &f_wall_distance) |
Examine way before the actor and return evasive direction (-1,+1) whenever there is need to evade the wall. Return 0 when no evasion is needed. | |
const bool | findInitialWay (void) |
Find safe initial walk direction. | |
void | avoidObstacle (AI_Obstacle *ptr_obstacle, const double d_time) |
Avoid particular obstacle. | |
void | avoidWall (const float f_dir, const double d_time) |
Avoid in given direction. | |
void | goBackToPath (const double d_time) |
When the actor is not on its way then this method turn them toward pivot. | |
void | doJink (const float f_evasive_angle) |
Change rotation by evasive angle. | |
bool | isDesiredAngle (const double d_time) |
Is actor in desired angle? | |
void | updateSteeringLengths (void) |
Update constants for reflexive behaviour. | |
void | makeDesiredAngleFromDirection (AI_Vector3 &v_direction) |
Compute desired angle of the actor from desired direction. | |
void | makeTurnDirection (void) |
Compute turn direction to desired angle (-1, 0, +1). | |
void | changeWayPoint (void) |
Move to the next way point in founded way. | |
bool | isFinishReached (const double d_time, AI_Obstacle *ptr_obstacle, const bool b_obstruct_dest) |
Is finish reached? | |
void | makePositionPrediction (AI_Vector3 &v_position, const double d_time) const |
Compute position prediction for given time. | |
void | sleep (const double d_time) |
Sleep actor for a while. | |
const bool | detectDeadWayWall (const float f_walk_evasive_direction, const float f_wall_distance) |
const bool | detectDeadWayObstacle (const double d_time, AI_Obstacle *ptr_obstacle, const float f_obstacle_distance) |
const bool | detectDeadWayFarFromPath (void) |
AI_Obstacle * | checkObstacleWhileWalking (AI_Obstacle *ptr_obstacle, const float f_obstacle_distance, bool &b_obstruct_dest) |
Check nearest obstacle and decide if it shoud be considered and how. | |
Classes | |
class | StateAct |
class | StateAttack |
class | StateChase |
class | StateIsAccessible |
class | StateIsAct |
class | StateIsFeedback |
class | StateIsHear |
State check if enemy is in earshot. More... | |
class | StateIsInOptimum |
class | StateIsInRange |
class | StateIsSee |
State checks if enemy is visible within sight and FOV. More... | |
class | StateIsTimePlan |
class | StateIsTouch |
class | StateMachineSession |
class | StateStop |
class | StateTimePlan |
class | StateWander |
Member Typedef Documentation
typedef AI_Array<AI_Actor*> AI_Actor::ActorArray |
Array of actor pointers.
Iterator of an array of actors.
Member Enumeration Documentation
enum AI_Actor::ACT |
enum AI_Actor::FEEDBACK |
enum AI_Actor::ENEMY_TYPE |
Constructor & Destructor Documentation
AI_Actor::AI_Actor | ( | void | ) |
Default constructor.
AI_Actor::~AI_Actor | ( | void | ) |
Destructor.
Member Function Documentation
void AI_Actor::trigger | ( | double | d_time | ) |
Trigger actor's behaviour in one frame.
Actor must be triggered in each frame to work properly. Once the actor is in AI world then it is triggered automatically when it is enabled.
- Parameters:
-
d_time Frame time
void AI_Actor::setLockedChased | ( | AI_Actor * | ptr_actor | ) | [inline] |
Lock actor for chasing.
AI_Actor * AI_Actor::getLockedChased | ( | void | ) | [inline] |
Get actor locked for chasing.
void AI_Actor::setLockedEnemy | ( | AI_Actor * | ptr_actor | ) | [inline] |
Lock an enemy.
void AI_Actor::setAttacker | ( | AI_Actor * | ptr_attacker | ) | [inline] |
Set an actor who attack this actor.
AI_Actor * AI_Actor::getLockedEnemy | ( | void | ) | [inline] |
Get locked enemy.
AI_Actor * AI_Actor::getAttacker | ( | void | ) | [inline] |
Get attacker.
bool AI_Actor::updateNearAgents | ( | const float | f_distance | ) |
Build array of agents that are nearer then given distance.
If time from last querry is more then some period constant and/or distance is longer then last retrieved distance then do querry into spatial database and retrieve near agents.
- Parameters:
-
f_distance Distance to what the agenst will be added into array.
- Returns:
- If new array of near agents was build then return true, otherwise return false.
AI_Agent::AgentArray & AI_Actor::getNearAgents | ( | const float | f_distance | ) | [inline] |
Return foreign agents in given range around this actor.
This array is cached for speed reasons. Array of agents is updated only once per period given by reflex value or imediately when some agents were added or removed form AI world or new distance is higher then previous distance. The last condition is reason why you must check distances of returned agents. Returned agents could be behind given distance.
Reimplemented from AI_Agent.
AI_Actor::ActorArray & AI_Actor::getNearActors | ( | const float | f_distance | ) |
Return foreign actors in given range around this actor.
This array is cached for speed reasons. Array of actors is updated only once per period given by reflex value or imediately when some agents were added or removed form AI world or new distance is higher then previous distance. The last condition is reason why you must check distances of returned actors. Returned actors could be behind given distance.
AI_Obstacle::ObstacleArray & AI_Actor::getNearObstacles | ( | const float | f_distance | ) |
Return foreign obstacles in given range around this actor.
This array is cached for speed reasons. Array of obstacles is updated only once per period given by reflex value or imediately when some agents were added or removed form AI world or new distance is higher then previous distance. The last condition is reason why you must check distances of returned obstacles. Returned actors could be behind given distance.
AI_Actor::ActorArray & AI_Actor::getNearEnemies | ( | const float | f_distance | ) |
const bool AI_Actor::isEnemy | ( | AI_Actor * | ptr_actor | ) |
Return true if given actor is an enemy for this actor.
void AI_Actor::setEnable | ( | const bool | b_enable | ) | [virtual] |
const AI_Vector3 & AI_Actor::getDestination | ( | void | ) | [inline] |
Return destination set previously by walkTo().
This method didn't return position of chased actor.
AI_NavigationMesh::Session & AI_Actor::getNavmeshSession | ( | void | ) | [inline] |
Get navigation mesh session.
void AI_Actor::clearWay | ( | void | ) | [inline] |
Clear any founded way.
int AI_Actor::getWaySize | ( | void | ) | const [inline] |
Number of waypoints on the way.
AI_Vector3 & AI_Actor::getWayPoint | ( | int | i | ) | const [inline] |
Get way point on the way (must be between 0 and way size).
const bool AI_Actor::isTouch | ( | AI_Obstacle * | ptr_agent_other, | |
const float | f_tolerance | |||
) | const [inline] |
Is actor touching another actor with given tolerance?
const bool AI_Actor::isCollision | ( | AI_Obstacle * | ptr_obstacle, | |
const double | d_time | |||
) | const [inline] |
Will the actor collide with given obstacle in given time?
The linear velocities of actors are considered.
const AI_Vector3 & AI_Actor::getWalkDirection | ( | void | ) | const [inline] |
Get vector of desired walking.
const AI_Vector3 & AI_Actor::getWalkWayPoint | ( | void | ) | const [inline] |
Get actual way point.
While actor is walking this is the nearest way point on its way.
const AI_Vector2 & AI_Actor::getFeelerTip | ( | void | ) | const [inline] |
Get feeler tip 2d position (for debug visualisation purposes).
const AI_Vector3 & AI_Actor::getPivot | ( | void | ) | const [inline] |
Get way pivot (for debug visualisation purposes).
const float AI_Actor::getTurnDirection | ( | void | ) | const [inline] |
Get turn direction.
Get direction to what the actor needs to be turning.
- -1 to the left
- +1 to the right
- 0 no turning
const float AI_Actor::getDesiredAngle | ( | void | ) | const [inline] |
Return desired angle.
const float AI_Actor::getWayLength | ( | void | ) | [inline] |
Overal length of the way.
bool AI_Actor::isOnSurface | ( | void | ) |
Is actor over surface of navigation mesh?
bool AI_Actor::getNearestSurfacePosition | ( | AI_Vector3 & | v_nearest_position | ) |
Return nearest position on navigation mesh to actor within range of his width.
void AI_Actor::setAIEnable | ( | bool | b_ai = true |
) | [inline] |
Enable/disable actor.
bool AI_Actor::isAIEnable | ( | void | ) | const [inline] |
Is AI enabled?
void AI_Actor::setStateMachine | ( | AI_StateMachine * | ptr_state_machine | ) | [inline] |
Set state machine which will drive this actor.
AI_StateMachine * AI_Actor::getStateMachine | ( | void | ) | [inline] |
Get state machine.
AI_StateMachine::Session & AI_Actor::getStateMachineSession | ( | void | ) | [inline] |
Get state machine session.
void AI_Actor::setAct | ( | int | i_act | ) | [inline] |
Set actual actor's acting.
int AI_Actor::getAct | ( | void | ) | const [inline] |
Return actual actor's acting.
const AI_Actor* AI_Actor::getAttackTarget | ( | void | ) |
const bool AI_Actor::isSleeping | ( | void | ) | const [inline] |
Return true if actor is sleeping (due to collision with another actor).
void AI_Actor::setFeelerLength | ( | bool | b_fixed, | |
float | f_length = -1 | |||
) | [inline] |
Set feeler length.
Set fixed or automatically update feeler length.
void AI_Actor::setPivotDistance | ( | bool | b_fixed, | |
float | f_distance = -1 | |||
) | [inline] |
Set pivot distance.
Set fixed or automatically updated pivot distance.
const float AI_Actor::getFeelerLength | ( | void | ) | const [inline] |
Get feeler length.
const float AI_Actor::getPivotDistance | ( | void | ) | const [inline] |
Get pivot distance.
void AI_Actor::setRefex | ( | const double | d_reflex | ) | [inline] |
Set time between two actor's reactions (in second).
void AI_Actor::setLinearVelocity | ( | const float | f_linear_velocity | ) | [inline] |
Set linear velocity.
void AI_Actor::setAngularVelocity | ( | const float | f_angular_velocity | ) | [inline] |
Set angular velocity.
void AI_Actor::setClan | ( | const int | i_clan | ) | [inline] |
Set clan into which the actor belongs.
void AI_Actor::setEnemyClan | ( | const int | i_clan | ) | [inline] |
Set enemy clan.
Considered only when ET_CLAN is set as enemy type;
void AI_Actor::setEnemyType | ( | const ENEMY_TYPE | type | ) | [inline] |
Set who is enemy for this actor.
void AI_Actor::setSight | ( | const float | f_sight | ) | [inline] |
Set maximum distance to what the actor can see other actors.
void AI_Actor::setEarshot | ( | const float | f_earshot | ) | [inline] |
Get maximum distance to what the actor can hear other actors.
void AI_Actor::setFOV | ( | const float | f_fov | ) | [inline] |
Set field of view (in radians).
void AI_Actor::setRange | ( | const float | f_range | ) | [inline] |
Set maximum distance for ranged attack.
void AI_Actor::setOptimalDistance | ( | const float | f_dist | ) | [inline] |
Set optimal distance for attack.
void AI_Actor::setChaseDistance | ( | const float | f_dist | ) | [inline] |
Set maximum distance for chasing.
void AI_Actor::setWidth | ( | const float | f_width | ) | [virtual] |
void AI_Actor::setVisible | ( | const bool | b | ) | [inline] |
Set actor's visibility.
void AI_Actor::setNoise | ( | const bool | b | ) | [inline] |
Set whether actor is performing some noise.
void AI_Actor::setUntouchable | ( | const bool | b | ) | [inline] |
Get pivot distance.
const float AI_Actor::getAngularVelocity | ( | void | ) | const [inline] |
Get angular velocity.
const double AI_Actor::getReflex | ( | void | ) | const [inline] |
Get reflex (in seconds).
const float AI_Actor::getLinearVelocity | ( | void | ) | const [inline] |
Get linear velocity of an actor.
const int AI_Actor::getClan | ( | void | ) | const [inline] |
Get clan into which the actor belongs.
const int AI_Actor::getEnemyClan | ( | void | ) | const [inline] |
Get enemy clan.
const AI_Actor::ENEMY_TYPE AI_Actor::getEnemyType | ( | void | ) | const [inline] |
Who is enemy for this actor?
const float AI_Actor::getSight | ( | void | ) | const [inline] |
Get maximum distance to what the actor can see other actors.
const float AI_Actor::getEarshot | ( | void | ) | const [inline] |
Get distance to what the acto hears other actors.
const float AI_Actor::getFOV | ( | void | ) | const [inline] |
Get field of view angle in radians.
const float AI_Actor::getRange | ( | void | ) | const [inline] |
Get maximum possible distance for attack.
const float AI_Actor::getOptimalDistance | ( | void | ) | const [inline] |
Get optimal distance for attack.
const float AI_Actor::getChaseDistance | ( | void | ) | const [inline] |
Get max chasing distance.
const bool AI_Actor::isVisible | ( | void | ) | const [inline] |
Is actor visible?
const bool AI_Actor::isNoise | ( | void | ) | const [inline] |
Is actor performing some noise?
const bool AI_Actor::isUntouchable | ( | void | ) | const [inline] |
Get pivot distance.
void AI_Actor::walkTo | ( | const AI_Vector3 & | v_dest | ) | [inline] |
Walk to given position.
Set destination and start walking or turning. Actor will keep walking until the destination is reached. Then he stops himself.
void AI_Actor::wander | ( | void | ) |
Wander.
void AI_Actor::turnTo | ( | const float | f_angle | ) | [inline] |
Turn to given angle.
Once the desired angle is set the actor starts turning and keep turning until he reach the desired angle.
void AI_Actor::chaseActor | ( | AI_Actor * | ptr_actor | ) |
Chase an actor.
Set or change already chased actor. Once the chased actor is set then this actor starts walking or turning and he will keep trying to walk towards the chased actor until he touch him. Then he stops himself.
void AI_Actor::attack | ( | AI_Actor * | ptr_actor | ) |
Attack another actor.
When the attack is finished then end of the attack feedback must be send.
void AI_Actor::stop | ( | void | ) | [inline] |
Instantly stops the actor whatever he is doing.
void AI_Actor::die | ( | void | ) | [inline] |
Kill actor.
Stop and set DEATH act.
void AI_Actor::reset | ( | void | ) | [inline] |
Reset actor.
Stop body, reset state machine and schedule state machine to work in next trigger() call.
void AI_Actor::sendFeedback | ( | FEEDBACK | dw_feedback | ) | [inline] |
Send feedback to this actor.
bool AI_Actor::checkFeedback | ( | ai_dword | dw_feedback | ) | [inline] |
Ask for particualar sent feedback.
void AI_Actor::clearFeedback | ( | ai_dword | dw_feedback | ) | [inline] |
Clear particular feedback.
void AI_Actor::flushFeedback | ( | void | ) | [inline] |
Clear all feedbacks.
void AI_Actor::updateWait | ( | const double | d_time | ) | [protected] |
Update waiting.
void AI_Actor::updateWalkDirection | ( | const double | d_time | ) | [protected] |
Update actor while he is walking.
void AI_Actor::updateWandering | ( | const double | d_time | ) | [protected] |
Update actor while wandering.
void AI_Actor::updateOrientation | ( | const double | d_time | ) | [protected] |
Update actor's orientation while actor is just turning.
Actor is "just turning" typically when he is going to walk.
void AI_Actor::updateAttack | ( | const double | d_time | ) | [protected] |
Update attacking.
bool AI_Actor::findWay | ( | void | ) | [protected] |
Instantly find way and return true if way was found.
void AI_Actor::updatePivot | ( | const double | d_time | ) | [protected] |
Return foreign items in given range around this actor.
This array is cached for speed reasons. Array of items is updated only once per period given by reflex value or imediately when some agents were added or removed form AI world or new distance is higher then previous distance. The last condition is reason why you must check distances of returned items. Returned actors could be behind given distance.
Move pivot along the way.
void AI_Actor::updateFeeler | ( | void | ) | [protected] |
Update feeler tip position.
void AI_Actor::updateDestination | ( | const double | d_time | ) | [protected] |
Update destination while some actor is chased.
AI_Obstacle * AI_Actor::findNearestObstacle | ( | const double | d_time, | |
float & | f_obstacle_distance | |||
) | [protected] |
Examine space before actor by feeler and return nearest feeled obstacle.
float AI_Actor::findNearestWall | ( | float & | f_wall_distance | ) | [protected] |
Examine way before the actor and return evasive direction (-1,+1) whenever there is need to evade the wall. Return 0 when no evasion is needed.
const bool AI_Actor::findInitialWay | ( | void | ) | [protected] |
Find safe initial walk direction.
Before the method is called then m_walk_direction must be set into ideal walk direction (path segment while walking, actor heading while wandering). If the method returns true then m_walk_direction is set as safe walk direction (without any obstacle or wall). The method changes m_walk_directiob event if it returns false.
This method divides ground near to an actor into hexagrams (one for an actor and six for surround) where one hexagram has same radius as an actor. Then hexagrams are checked for walk safety started from hexagram in ideal walk direction and ended by hexagrams oposite to ideal walk direction. Hexagrams are checked until the safe walk direction is found or until no safe way is detected.
- Returns:
- true when safe walk direction is found.
void AI_Actor::avoidObstacle | ( | AI_Obstacle * | ptr_obstacle, | |
const double | d_time | |||
) | [protected] |
Avoid particular obstacle.
- Parameters:
-
ptr_obstacle Obstacle to avoid d_time Frame time
void AI_Actor::avoidWall | ( | const float | f_dir, | |
const double | d_time | |||
) | [inline, protected] |
Avoid in given direction.
- Parameters:
-
f_dir Direction (-1,0,+1) d_time Frame time
void AI_Actor::goBackToPath | ( | const double | d_time | ) | [protected] |
When the actor is not on its way then this method turn them toward pivot.
void AI_Actor::doJink | ( | const float | f_evasive_angle | ) | [inline, protected] |
Change rotation by evasive angle.
bool AI_Actor::isDesiredAngle | ( | const double | d_time | ) | [inline, protected] |
Is actor in desired angle?
Desired angle is comupted before turning is started.
void AI_Actor::updateSteeringLengths | ( | void | ) | [inline, protected] |
Update constants for reflexive behaviour.
void AI_Actor::makeDesiredAngleFromDirection | ( | AI_Vector3 & | v_direction | ) | [inline, protected] |
Compute desired angle of the actor from desired direction.
void AI_Actor::makeTurnDirection | ( | void | ) | [inline, protected] |
Compute turn direction to desired angle (-1, 0, +1).
void AI_Actor::changeWayPoint | ( | void | ) | [inline, protected] |
Move to the next way point in founded way.
bool AI_Actor::isFinishReached | ( | const double | d_time, | |
AI_Obstacle * | ptr_obstacle, | |||
const bool | b_obstruct_dest | |||
) | [protected] |
Is finish reached?
Return true when actor reached the destination set by walkTo or touch the obstacle which obstruct the the destination.
void AI_Actor::makePositionPrediction | ( | AI_Vector3 & | v_position, | |
const double | d_time | |||
) | const [inline, protected] |
Compute position prediction for given time.
Consider actual position, linera velocity (when walking) and given time.
void AI_Actor::sleep | ( | const double | d_time | ) | [inline, protected] |
Sleep actor for a while.
Used when actor is walking.
const bool AI_Actor::detectDeadWayWall | ( | const float | f_walk_evasive_direction, | |
const float | f_wall_distance | |||
) | [protected] |
const bool AI_Actor::detectDeadWayObstacle | ( | const double | d_time, | |
AI_Obstacle * | ptr_obstacle, | |||
const float | f_obstacle_distance | |||
) | [protected] |
const bool AI_Actor::detectDeadWayFarFromPath | ( | void | ) | [protected] |
AI_Obstacle * AI_Actor::checkObstacleWhileWalking | ( | AI_Obstacle * | ptr_obstacle, | |
const float | f_obstacle_distance, | |||
bool & | b_obstruct_dest | |||
) | [protected] |
Check nearest obstacle and decide if it shoud be considered and how.
If obstacle need to be considered then pointer to obstacle is returned back. If obstacle need to be considered and obstruct destination point then b_obstruct_dest is set to true.
Member Data Documentation
AI agent is enabled.
There are new founded agents that weren't transfered into obstacles array.
There are new founded agents that weren't transfered into actors array.
There are new founded agents that weren't transfered into items array.
There are new founded agents that weren't transfered into enemies array.
Walking actor is on path.
Actor is heading to pivot.
It is need to replan path.
Actual act is ATTACK but one attack was finished.
bool AI_Actor::m_b_sleep |
Actor is walking but now it sleeps for a while from some reason.
Actor is visible.
bool AI_Actor::m_b_noise |
Actor is performing some noise.
Fixed feeler length (feeler length is set by user).
Fixed pivot distance (pivot distance is set by user).
This actor in untouchable (can't be attacked).
The documentation for this class was generated from the following files: